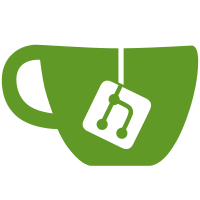
This repository contains Transmission RSS Manager with the following changes: - Fixed dark mode navigation tab visibility issue - Improved text contrast in dark mode throughout the app - Created dedicated dark-mode.css for better organization - Enhanced JavaScript for dynamic styling in dark mode - Added complete installation scripts 🤖 Generated with [Claude Code](https://claude.ai/code) Co-Authored-By: Claude <noreply@anthropic.com>
400 lines
14 KiB
C#
400 lines
14 KiB
C#
// <auto-generated />
|
|
using System;
|
|
using Microsoft.EntityFrameworkCore;
|
|
using Microsoft.EntityFrameworkCore.Infrastructure;
|
|
using Microsoft.EntityFrameworkCore.Storage.ValueConversion;
|
|
using Npgsql.EntityFrameworkCore.PostgreSQL.Metadata;
|
|
using TransmissionRssManager.Data;
|
|
|
|
#nullable disable
|
|
|
|
namespace TransmissionRssManager.Migrations
|
|
{
|
|
[DbContext(typeof(TorrentManagerContext))]
|
|
partial class TorrentManagerContextModelSnapshot : ModelSnapshot
|
|
{
|
|
protected override void BuildModel(ModelBuilder modelBuilder)
|
|
{
|
|
#pragma warning disable 612, 618
|
|
modelBuilder
|
|
.HasAnnotation("ProductVersion", "7.0.17")
|
|
.HasAnnotation("Relational:MaxIdentifierLength", 63);
|
|
|
|
NpgsqlModelBuilderExtensions.UseIdentityByDefaultColumns(modelBuilder);
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.RssFeed", b =>
|
|
{
|
|
b.Property<int>("Id")
|
|
.ValueGeneratedOnAdd()
|
|
.HasColumnType("integer");
|
|
|
|
NpgsqlPropertyBuilderExtensions.UseIdentityByDefaultColumn(b.Property<int>("Id"));
|
|
|
|
b.Property<DateTime>("CreatedAt")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<string>("DefaultCategory")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<bool>("Enabled")
|
|
.HasColumnType("boolean");
|
|
|
|
b.Property<int>("ErrorCount")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<DateTime>("LastCheckedAt")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<string>("LastError")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<int>("MaxHistoryItems")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<string>("Name")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<int>("RefreshInterval")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<string>("Schedule")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<string>("TransmissionInstanceId")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<DateTime?>("UpdatedAt")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<string>("Url")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.HasKey("Id");
|
|
|
|
b.ToTable("RssFeeds");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.RssFeedItem", b =>
|
|
{
|
|
b.Property<int>("Id")
|
|
.ValueGeneratedOnAdd()
|
|
.HasColumnType("integer");
|
|
|
|
NpgsqlPropertyBuilderExtensions.UseIdentityByDefaultColumn(b.Property<int>("Id"));
|
|
|
|
b.Property<string>("Description")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<DateTime>("DiscoveredAt")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<string>("DownloadError")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<DateTime?>("DownloadedAt")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<bool>("IsDownloaded")
|
|
.HasColumnType("boolean");
|
|
|
|
b.Property<string>("Link")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<int?>("MatchedRuleId")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<DateTime>("PublishDate")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<int>("RssFeedId")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<string>("Title")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<int?>("TorrentId")
|
|
.HasColumnType("integer");
|
|
|
|
b.HasKey("Id");
|
|
|
|
b.HasIndex("MatchedRuleId");
|
|
|
|
b.HasIndex("RssFeedId", "Link")
|
|
.IsUnique();
|
|
|
|
b.ToTable("RssFeedItems");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.RssFeedRule", b =>
|
|
{
|
|
b.Property<int>("Id")
|
|
.ValueGeneratedOnAdd()
|
|
.HasColumnType("integer");
|
|
|
|
NpgsqlPropertyBuilderExtensions.UseIdentityByDefaultColumn(b.Property<int>("Id"));
|
|
|
|
b.Property<DateTime>("CreatedAt")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<string>("CustomSavePath")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<bool>("EnablePostProcessing")
|
|
.HasColumnType("boolean");
|
|
|
|
b.Property<bool>("Enabled")
|
|
.HasColumnType("boolean");
|
|
|
|
b.Property<string>("ExcludePattern")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<string>("IncludePattern")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<string>("Name")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<int>("Priority")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<int>("RssFeedId")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<bool>("SaveToCustomPath")
|
|
.HasColumnType("boolean");
|
|
|
|
b.Property<DateTime?>("UpdatedAt")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<bool>("UseRegex")
|
|
.HasColumnType("boolean");
|
|
|
|
b.HasKey("Id");
|
|
|
|
b.HasIndex("RssFeedId");
|
|
|
|
b.ToTable("RssFeedRules");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.SystemLogEntry", b =>
|
|
{
|
|
b.Property<int>("Id")
|
|
.ValueGeneratedOnAdd()
|
|
.HasColumnType("integer");
|
|
|
|
NpgsqlPropertyBuilderExtensions.UseIdentityByDefaultColumn(b.Property<int>("Id"));
|
|
|
|
b.Property<string>("Context")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<string>("Level")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<string>("Message")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<string>("Properties")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<DateTime>("Timestamp")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.HasKey("Id");
|
|
|
|
b.ToTable("SystemLogs");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.Torrent", b =>
|
|
{
|
|
b.Property<int>("Id")
|
|
.ValueGeneratedOnAdd()
|
|
.HasColumnType("integer");
|
|
|
|
NpgsqlPropertyBuilderExtensions.UseIdentityByDefaultColumn(b.Property<int>("Id"));
|
|
|
|
b.Property<DateTime>("AddedOn")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<string>("Category")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<DateTime?>("CompletedOn")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<string>("DownloadDirectory")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<double>("DownloadSpeed")
|
|
.HasColumnType("double precision");
|
|
|
|
b.Property<long>("DownloadedEver")
|
|
.HasColumnType("bigint");
|
|
|
|
b.Property<string>("ErrorMessage")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<bool>("HasMetadata")
|
|
.HasColumnType("boolean");
|
|
|
|
b.Property<string>("Hash")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<string>("Name")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<int>("PeersConnected")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<double>("PercentDone")
|
|
.HasColumnType("double precision");
|
|
|
|
b.Property<bool>("PostProcessed")
|
|
.HasColumnType("boolean");
|
|
|
|
b.Property<DateTime?>("PostProcessedOn")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<int?>("RssFeedItemId")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<string>("Status")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<long>("TotalSize")
|
|
.HasColumnType("bigint");
|
|
|
|
b.Property<int?>("TransmissionId")
|
|
.HasColumnType("integer");
|
|
|
|
b.Property<string>("TransmissionInstance")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<double>("UploadRatio")
|
|
.HasColumnType("double precision");
|
|
|
|
b.Property<double>("UploadSpeed")
|
|
.HasColumnType("double precision");
|
|
|
|
b.Property<long>("UploadedEver")
|
|
.HasColumnType("bigint");
|
|
|
|
b.HasKey("Id");
|
|
|
|
b.HasIndex("Hash")
|
|
.IsUnique();
|
|
|
|
b.HasIndex("RssFeedItemId")
|
|
.IsUnique();
|
|
|
|
b.ToTable("Torrents");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.UserPreference", b =>
|
|
{
|
|
b.Property<int>("Id")
|
|
.ValueGeneratedOnAdd()
|
|
.HasColumnType("integer");
|
|
|
|
NpgsqlPropertyBuilderExtensions.UseIdentityByDefaultColumn(b.Property<int>("Id"));
|
|
|
|
b.Property<string>("Category")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<DateTime>("CreatedAt")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<string>("DataType")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<string>("Description")
|
|
.HasColumnType("text");
|
|
|
|
b.Property<string>("Key")
|
|
.IsRequired()
|
|
.HasColumnType("text");
|
|
|
|
b.Property<DateTime?>("UpdatedAt")
|
|
.HasColumnType("timestamp with time zone");
|
|
|
|
b.Property<string>("Value")
|
|
.HasColumnType("text");
|
|
|
|
b.HasKey("Id");
|
|
|
|
b.HasIndex("Key")
|
|
.IsUnique();
|
|
|
|
b.ToTable("UserPreferences");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.RssFeedItem", b =>
|
|
{
|
|
b.HasOne("TransmissionRssManager.Data.Models.RssFeedRule", "MatchedRule")
|
|
.WithMany("MatchedItems")
|
|
.HasForeignKey("MatchedRuleId")
|
|
.OnDelete(DeleteBehavior.SetNull);
|
|
|
|
b.HasOne("TransmissionRssManager.Data.Models.RssFeed", "RssFeed")
|
|
.WithMany("Items")
|
|
.HasForeignKey("RssFeedId")
|
|
.OnDelete(DeleteBehavior.Cascade)
|
|
.IsRequired();
|
|
|
|
b.Navigation("MatchedRule");
|
|
|
|
b.Navigation("RssFeed");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.RssFeedRule", b =>
|
|
{
|
|
b.HasOne("TransmissionRssManager.Data.Models.RssFeed", "RssFeed")
|
|
.WithMany("Rules")
|
|
.HasForeignKey("RssFeedId")
|
|
.OnDelete(DeleteBehavior.Cascade)
|
|
.IsRequired();
|
|
|
|
b.Navigation("RssFeed");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.Torrent", b =>
|
|
{
|
|
b.HasOne("TransmissionRssManager.Data.Models.RssFeedItem", "RssFeedItem")
|
|
.WithOne("Torrent")
|
|
.HasForeignKey("TransmissionRssManager.Data.Models.Torrent", "RssFeedItemId")
|
|
.OnDelete(DeleteBehavior.SetNull);
|
|
|
|
b.Navigation("RssFeedItem");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.RssFeed", b =>
|
|
{
|
|
b.Navigation("Items");
|
|
|
|
b.Navigation("Rules");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.RssFeedItem", b =>
|
|
{
|
|
b.Navigation("Torrent");
|
|
});
|
|
|
|
modelBuilder.Entity("TransmissionRssManager.Data.Models.RssFeedRule", b =>
|
|
{
|
|
b.Navigation("MatchedItems");
|
|
});
|
|
#pragma warning restore 612, 618
|
|
}
|
|
}
|
|
}
|