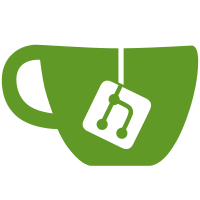
This repository contains Transmission RSS Manager with the following changes: - Fixed dark mode navigation tab visibility issue - Improved text contrast in dark mode throughout the app - Created dedicated dark-mode.css for better organization - Enhanced JavaScript for dynamic styling in dark mode - Added complete installation scripts 🤖 Generated with [Claude Code](https://claude.ai/code) Co-Authored-By: Claude <noreply@anthropic.com>
122 lines
2.9 KiB
Bash
Executable File
122 lines
2.9 KiB
Bash
Executable File
#\!/bin/bash
|
|
|
|
# This module handles configuration related tasks
|
|
|
|
create_default_config() {
|
|
local config_file=$1
|
|
|
|
# Check if config file already exists
|
|
if [ -f "$config_file" ]; then
|
|
echo "Configuration file already exists at $config_file"
|
|
return 0
|
|
fi
|
|
|
|
echo "Creating default configuration..."
|
|
|
|
# Create default appsettings.json
|
|
cat > "$config_file" << EOL
|
|
{
|
|
"Logging": {
|
|
"LogLevel": {
|
|
"Default": "Information",
|
|
"Microsoft.AspNetCore": "Warning"
|
|
}
|
|
},
|
|
"AllowedHosts": "*",
|
|
"DatabaseSettings": {
|
|
"ConnectionString": "Data Source=torrentmanager.db",
|
|
"UseInMemoryDatabase": true
|
|
},
|
|
"Serilog": {
|
|
"MinimumLevel": {
|
|
"Default": "Information",
|
|
"Override": {
|
|
"Microsoft": "Warning",
|
|
"System": "Warning"
|
|
}
|
|
},
|
|
"WriteTo": [
|
|
{
|
|
"Name": "Console",
|
|
"Args": {
|
|
"outputTemplate": "[{Timestamp:HH:mm:ss} {Level:u3}] {Message:lj}{NewLine}{Exception}"
|
|
}
|
|
},
|
|
{
|
|
"Name": "File",
|
|
"Args": {
|
|
"path": "logs/log-.txt",
|
|
"rollingInterval": "Day",
|
|
"outputTemplate": "[{Timestamp:yyyy-MM-dd HH:mm:ss.fff zzz} {Level:u3}] {Message:lj}{NewLine}{Exception}"
|
|
}
|
|
}
|
|
]
|
|
},
|
|
"AppConfig": {
|
|
"Transmission": {
|
|
"Host": "localhost",
|
|
"Port": 9091,
|
|
"UseHttps": false,
|
|
"Username": "",
|
|
"Password": ""
|
|
},
|
|
"AutoDownloadEnabled": false,
|
|
"CheckIntervalMinutes": 30,
|
|
"DownloadDirectory": "",
|
|
"MediaLibraryPath": "",
|
|
"PostProcessing": {
|
|
"Enabled": false,
|
|
"ExtractArchives": true,
|
|
"OrganizeMedia": true,
|
|
"MinimumSeedRatio": 1,
|
|
"MediaExtensions": [
|
|
".mp4",
|
|
".mkv",
|
|
".avi"
|
|
],
|
|
"AutoOrganizeByMediaType": true,
|
|
"RenameFiles": false,
|
|
"CompressCompletedFiles": false,
|
|
"DeleteCompletedAfterDays": 0
|
|
},
|
|
"EnableDetailedLogging": false,
|
|
"UserPreferences": {
|
|
"EnableDarkMode": false,
|
|
"AutoRefreshUIEnabled": true,
|
|
"AutoRefreshIntervalSeconds": 30,
|
|
"NotificationsEnabled": true,
|
|
"NotificationEvents": [
|
|
"torrent-added",
|
|
"torrent-completed",
|
|
"torrent-error"
|
|
],
|
|
"DefaultView": "dashboard",
|
|
"ConfirmBeforeDelete": true,
|
|
"MaxItemsPerPage": 25,
|
|
"DateTimeFormat": "yyyy-MM-dd HH:mm:ss",
|
|
"ShowCompletedTorrents": true,
|
|
"KeepHistoryDays": 30
|
|
}
|
|
}
|
|
}
|
|
EOL
|
|
|
|
echo "Default configuration created"
|
|
}
|
|
|
|
update_config_value() {
|
|
local config_file=$1
|
|
local key=$2
|
|
local value=$3
|
|
|
|
# This is a simplified version that assumes jq is installed
|
|
# For a production script, you might want to add error checking
|
|
if command -v jq &> /dev/null; then
|
|
tmp=$(mktemp)
|
|
jq ".$key = \"$value\"" "$config_file" > "$tmp" && mv "$tmp" "$config_file"
|
|
echo "Updated $key to $value"
|
|
else
|
|
echo "jq is not installed, skipping config update"
|
|
fi
|
|
}
|